Reflective DLL Injection is one of those topics that caught my interest while digging through different malware behaviors in Windows environments. It’s not just a theoretical concept—it’s actively used by red teamers and malicious actors alike to stealthily load code into memory without touching disk. What intrigued me most is its elegance: bypassing traditional loading mechanisms, dodging antivirus software, and leaving minimal forensic traces.
In this blog, I’ll break down what Reflective DLL Injection is, how it works under the hood, and walk through how I implemented and detected it in a Windows lab environment. I’ll also touch on platform-specific considerations, detection strategies, and relevant code samples.
Table of Contents
What Is Reflective DLL Injection?
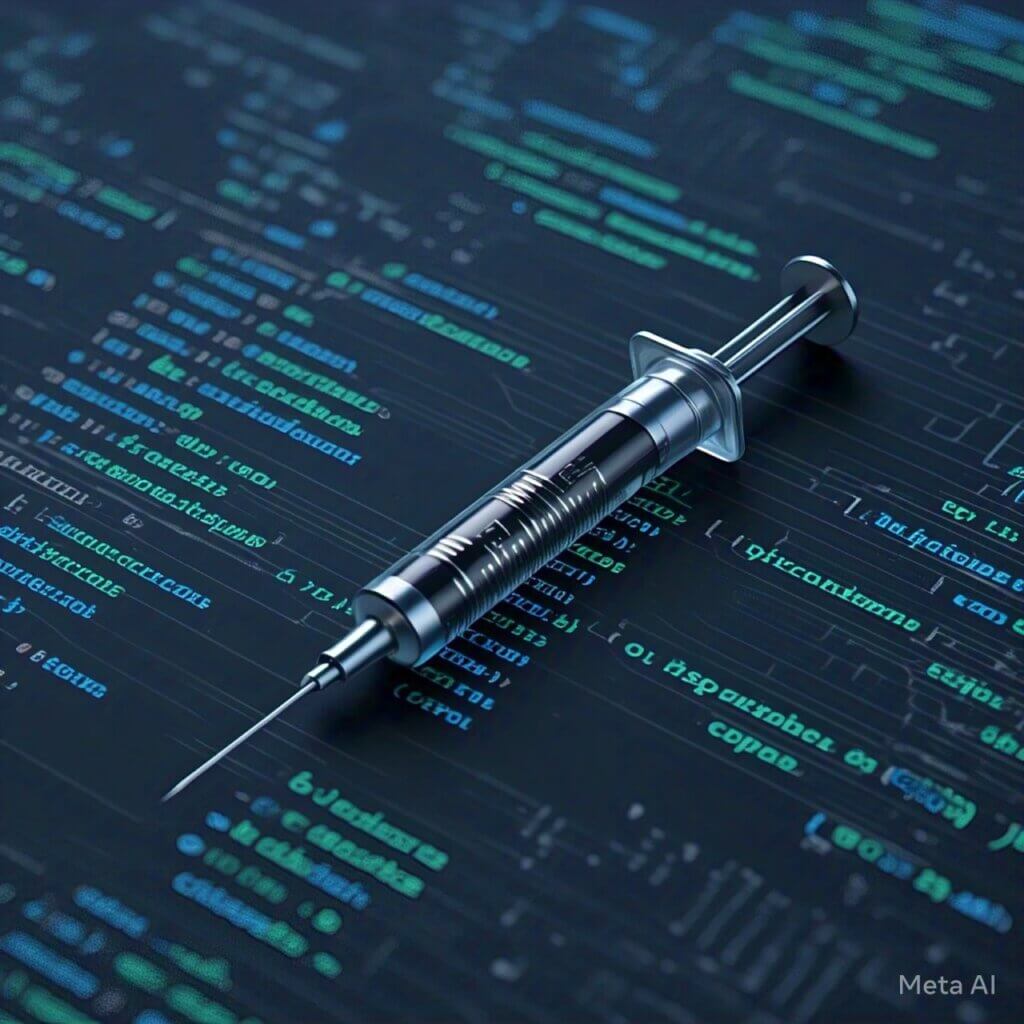
Reflective DLL Injection is a technique used to inject a Dynamic Link Library (DLL) into the address space of a process and execute it without relying on Windows APIs like LoadLibrary
. Instead, the DLL is loaded and executed entirely from memory. This is commonly used by advanced persistent threats (APTs), red team tools like Cobalt Strike, and some sophisticated malware strains.
The core idea is simple: you write a loader (called a reflective loader) inside the DLL itself that maps the DLL into memory, resolves imports, applies relocations, and jumps to the entry point. You then inject this DLL as a byte array into the remote process and call the reflective loader.
Key Steps in Reflective DLL Injection
Compile a DLL that includes a reflective loader.
Read the DLL as a byte array in the injector process.
Allocate memory in the target process.
Write the DLL into the target process’s memory.
Create a remote thread pointing to the reflective loader’s address.
How I Implemented Reflective DLL Injection
I began with a simple DLL that I wanted to inject. This DLL includes the reflective loader, which is a custom entry point responsible for loading the DLL manually.
Reflective DLL Sample (Simplified)
Here’s a simplified outline of the ReflectiveLoader function inside the DLL:
DWORD WINAPI ReflectiveLoader(void* lpParameter) {
// Parse the PE headers
// Allocate memory for the new image
// Copy sections into new memory
// Resolve imports manually
// Handle relocations
// Call DllMain
return 0;
}
In a real-world scenario, I used Stephen Fewer’s open-source reflective loader from the Metasploit framework and tailored it to my needs.
Injector Code (C++)
This is the injector application I used to inject the DLL into a remote process:
void InjectDLL(HANDLE hProcess, LPVOID dllBuffer, DWORD dllLength) {
LPVOID remoteMemory = VirtualAllocEx(hProcess, NULL, dllLength, MEM_COMMIT, PAGE_EXECUTE_READWRITE);
WriteProcessMemory(hProcess, remoteMemory, dllBuffer, dllLength, NULL);
// Calculate offset to ReflectiveLoader
DWORD offset = GetReflectiveLoaderOffset(dllBuffer);
LPTHREAD_START_ROUTINE pThreadStart = (LPTHREAD_START_ROUTINE)((LPBYTE)remoteMemory + offset);
HANDLE hThread = CreateRemoteThread(hProcess, NULL, 0, pThreadStart, remoteMemory, 0, NULL);
WaitForSingleObject(hThread, INFINITE);
}
This code reads a DLL file into memory, writes it into the target process, and launches a thread at the reflective loader’s entry point.
Why Reflective DLL Injection Works So Well
Traditional DLL injection leaves traces: the DLL shows up in memory-mapped files, the import table is populated, and it may appear in API hooks. Reflective DLL injection avoids all this because it doesn’t use LoadLibrary
, doesn’t touch disk, and doesn’t register with standard Windows APIs.
The injected code only lives in memory and the process’s Import Address Table (IAT) never knows it exists. It’s stealthy by design.
Platform-Specific Detection: Windows
To detect Reflective DLL Injection on Windows, I had to step away from API-based monitoring and instead dig directly into process memory. Here’s how I approached it:
1. Scanning Memory for Unbacked PE Files
I used PE-sieve to scan the memory of suspect processes. PE-sieve can detect PE files that are loaded in memory but don’t exist on disk.
pe-sieve64.exe /pid 1234 /mem_scan /dir output
This quickly helped me identify manually mapped DLLs that shouldn’t have been there. Reflective DLLs stand out because they lack file path references.
2. YARA Rule for PE Headers in Memory
Here’s a sample YARA rule I wrote to find PE headers in memory:
rule SuspiciousReflectivePE
{
strings:
$mz = { 4D 5A } // MZ Header
$pe = { 50 45 00 00 } // PE00 Header
condition:
$mz at 0 and $pe in (0..512)
}
Running this against memory dumps helped locate suspicious PE headers that weren’t tied to legitimate DLLs.
3. ETW + Sysmon Correlation
Since reflective DLLs don’t trigger LoadLibrary
events, I configured Sysmon to log memory allocations and remote thread creation. I correlated these with ETW events to find processes that had code injected but didn’t show any module load events.
<Sysmon>
<EventFiltering>
<RuleGroup name="Code Injection" groupRelation="or">
<Rule name="Load Library Bypass" condition="image">*\rundll32.exe</Rule>
<Rule name="Remote Thread Creation" condition="eventID">8</Rule>
</RuleGroup>
</EventFiltering>
</Sysmon>
Reflective DLL Injection in Cobalt Strike
When I explored Cobalt Strike’s beacons, I noticed it uses Reflective DLL Injection heavily. The beacons are staged payloads loaded directly into memory. If you analyze the process memory, you’ll see reflective DLLs that never touched the disk.
One of the simplest ways to catch this in an enterprise is to use behavioral detection. If a process suddenly allocates memory with PAGE_EXECUTE_READWRITE and spawns a thread from that region, you’re probably looking at reflective code loading.
Defenses and Hardening
There are a few ways to make your environment less vulnerable:
Use Application Whitelisting (e.g., AppLocker or WDAC)
Enable Windows Defender Exploit Guard with ASR rules to block behaviors like suspicious memory allocations and process injections
Deploy EDRs that do memory-level inspection, not just file scanning
Regularly scan with tools like PE-sieve or dump memory with Volatility for offline analysis
Final Thoughts
Reflective DLL Injection is elegant and dangerous. It’s a testament to how attackers can bypass traditional defenses with a deep understanding of how Windows works. I’ve used this technique in red teaming to simulate real-world malware, and every time I’m impressed by how subtle it can be.
If you’re responsible for detecting or defending Windows systems, understanding reflective injection is no longer optional. It’s foundational. By combining memory analysis, event logging, and proactive hunting, you can stay ahead of attackers who rely on memory-based stealth.
References
Reflective DLL Injection- Stephen Fewer, Github
Why Businesses Trust SecureMyOrg for Comprehensive Network Security
At SecureMyOrg, we uncover and fix all possible security vulnerabilities of mobile and web, while providing solutions to mitigate risks. We are trusted by renowned companies like Yahoo, Gojek and Rippling, and with 100% client satisfaction, you’re in safe hands!
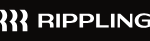
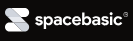
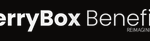
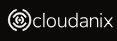
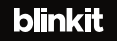
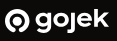

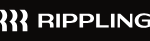
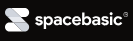
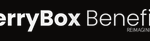
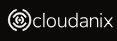
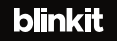
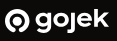
Some of the things people reach out to us for –
- Building their cybersecurity program from scratch – setting up cloud security using cost-effective tools, SIEM for alert monitoring, building policies for the company
- Vulnerability Assessment and Penetration Testing ( VAPT ) – We have certified professionals, with certifications like OSCP, CREST – CPSA & CRT, CKA and CKS
- DevSecOps consulting
- Red Teaming activity
- Regular security audits, before product release
- Full time security engineers.
Relevant Posts
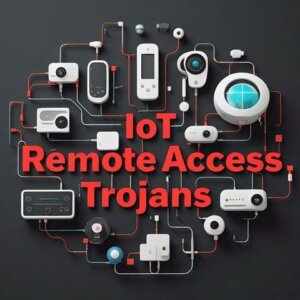
Top 5 IoT Remote Access Trojans Crippling Devices in 2025
IoT devices are under siege in 2025 as Remote Access Trojans exploit their vulnerabilities at scale. This blog breaks down the top 5 IoT RATs causing widespread disruption.
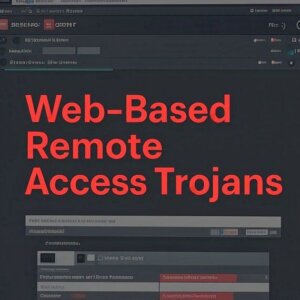
Top 5 Web-Based Remote Access Trojans That Are Dominating 2025
Web-based Remote Access Trojans are becoming the go-to tool for cybercriminals in 2025. This post highlights five of the most widespread and dangerous ones currently in use.
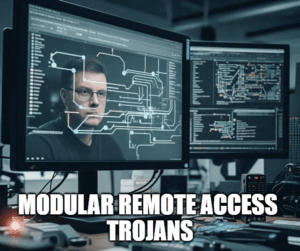
Unstoppable Malware: Top 5 Modular Remote Access Trojans Dominating 2025
Modular Remote Access Trojans are evolving fast in 2025, making them harder to detect and remove. This post explores five of the most dangerous RATs currently used in cyberattacks.
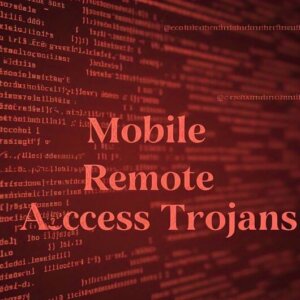
Top 5 Mobile Remote Access Trojans Wreaking Havoc in 2025
Uncover the top 5 mobile RATs of 2025, learn how they infect devices, execute attacks, and discover key strategies to detect and stop them effectively.
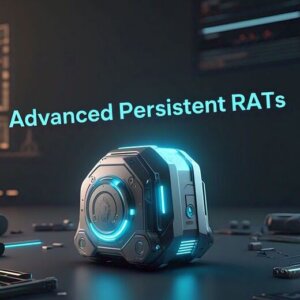
Top 5 Advanced Persistent Remote Access Trojans (RATs) in 2025
This blog explores five of the most sophisticated Advanced Persistent Remote Access Trojans (AP-RATs) currently active in the cyber threat landscape. We analyze their infection vectors, stealth mechanisms, command-and-control infrastructure, and persistence techniques to help security professionals understand and defend against these high-risk threats.
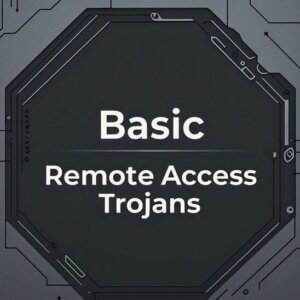
Top 5 Basic Remote Access Trojans (RATs) You Shouldn’t Ignore in 2025
Remote Access Trojans (RATs) remain a major cybersecurity threat in 2025. Learn about the top 5 basic yet dangerous RATs known for stealthy infiltration, keylogging, and full system control. Learn how they operate and how to defend against them.